
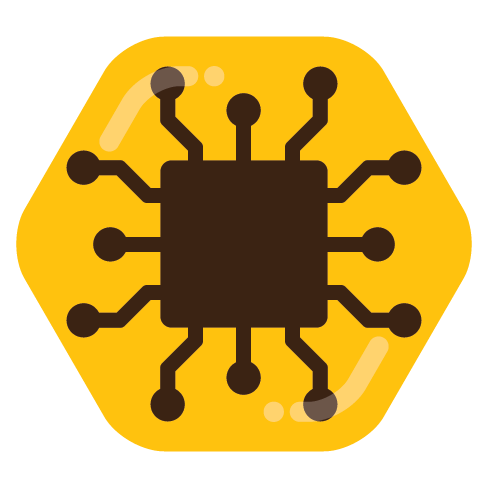
The key search term is “userChrome” (userChrome.css and userChrome.js) and XUL, which is the HTML-like language FF uses to define its chrome. “Chrome” is a term that predates Google’s browser, referring to the interface surrounding the displayed web content and Firefox still uses that internally.
Right now mine is pretty minimal, but there’s a lot you can change. Essentially, the interface is a kind of HTML page which can use the same features as normal HTML and can even run custom JavaScript. Also look into BetterFox for how to remove Mozilla’s own telemetry and bloat.
My userChrome.css for reference;
spoiler
/* Move findbar to the top */
.browserContainer > findbar {
-moz-box-ordinal-group:0 !important; /* for 112 and older */
order: -1 !important; /* for 113 and newer */
border-top: none !important;
border-bottom: 1px solid ThreeDShadow !important;
}
/* Remove "Open All In Tabs" button in bookmarks folders */
#PlacesToolbarItems .openintabs-menuitem,
#placesContext>menuitem[id="placesContext_openContainer:tabs"],
#placesContext>menuitem[id="placesContext_openContainer:tabs"]:not([hidden])+menuitem+#placesContext_openSeparator {
visibility: collapse !important;
}
/* Tabs are attached on the bottom */
.tab-background {
border-radius: var(--tab-border-radius) var(--tab-border-radius) 0 0 !important;
margin-top: 1px !important;
margin-bottom: 0 !important;
padding-bottom: 31px !important;
}
.tabbrowser-tab[multiselected=true]:not([selected=true]) .tab-background {
border-radius: var(--tab-border-radius) !important;
margin-top: 2px !important;
margin-bottom: 1px !important;
padding-bottom: 29px !important;
}
.tabbrowser-tab[selected=true] .tab-background ,
.tabbrowser-tab[multiselected=true] .tab-background {
background-color: var(--toolbar-bgcolor) !important;
background-image: var(--toolbar-bgimage) !important;
}
I found this repo which is supposed to apply Chromium styles in line with Google’s Material Design guidelines.
Here’s an article I found with some simple tweaks.
I have dozens of projects in varying levels of completion and maybe like 2 finished projects. Here’s my list, steal to your liking because I come up with ideas I want to see in the world, and clearly I’m not a great medium for that:
d(0, 255) = 1
, and it still worked. Stochastic updates work, even in a distributed context, because it’s a kind of “simulated annealing”.And finally then there’s my magnum opus, Espresso, my favorite project I keep coming back to time and time again and
bikesheddingrefining over many years. If anyone else takes it up I’d be ecstatic.proto[...T] Super Sub(...args) { ... }
, egproto class Animal { ... }
andproto Animal Monkey { ... }
class
,enum
,union
,interface
,struct
, etc. Compare to [P0707];
is an optional operator to explicitly disambiguate their ending.after
operator,x() after y() == (var t = x(); y(); t)
- surprisingly useful for conciseness.[...for(var x in 10) x] == list(range(10))
proto class { +(rhs) { console.log("\{this} + \{rhs}"); } }
delegate()
method which define how to represent the variable on the stack. Untyped variables use an inferred type or an implicitany
, which wraps a dynamic object. This lets you create objects likeint32
while still using the prototype semantics.unsafe
compilation distinction to avoid catastrophic vulnerabilities.try!
semantics, exceptions are actually wrapped in a returnedResult[T, E]
type:try
is an operator which unwraps the result and returns if it’s an error. Thus you getvar value = try can_fail();
. Using type object operator overloading, theResult
type doesn’t need to be explicitly annotated becauseResult[T, E] == T | Result[T, E] == T | fail E
.fail
keyword instead ofthrow
/raise
.I could go on for hours with all of the stuff I’ve thought of for this language. If you want to know more, the README.md and ideas.md are usually the most authoritative, and specification.md is a very formal description of a subset of the stuff that is absolutely 100% decided (mostly syntax). I’ve written turing complete subsets of it before. First usable implementation to arrive by sometime in 2200 lmao. 🫠 I can also unpack the other projects if you want to know more.